Importing Salesloft Opportunities via API
Introduction
Salesloft allows customers without a configured CRM to create and manage opportunities via API. Below is a guide to using these API endpoints, covering key operations like creating opportunities, managing stages, and person association.
Important Notes:
- Salesloft opportunities created via API will not be shown in Deals. They will appear on the Account and Person pages.
- Salesloft customers using Salesforce, Dynamics or Hubspot can only read Opportunity data via API. All updates must be done in the CRM or Deals platform.
Supported Users
- Salesloft customers with custom/alternate CRMs
Prerequisites
- API Authentication: You will need to authenticate API requests using an API key or OAuth application. Ensure you have a valid API key or token.
- Base URL:
https://api.salesloft.com/
Create an Opportunity Configuration
Salesloft customers without a configured CRM will register their applications with Salesloft by creating an external ID configuration. Learn more about external ids here.
Endpoint: POST v2/external/configurations
Request Body
{
"external_type": "crm_opportunity",
"object_type": "opportunities"
}
The external_type
field can be any name that aligns with your business or tech stack.
Create Opportunity Stages
To create opportunities in Salesloft, you need valid Opportunity Stages.
Endpoint: POST v2/opportunity_stages
Request Body
{
"name": "Evaluation",
"order": 1
}
Salesloft doesn’t require a stage to be tied to a probability or the closed/won booleans on the Opportunity resource. You will need to handle these validations in your codebase.
Create Opportunities
A Salesloft account_id is required to create opportunities. Opportunities are created and updated using an Upsert operation with an external ID.
Note: By default, opportunities created without an owner_id will be assigned to the user associated with the API key or auth token.
Upsert Endpoint: POST v2/external/ids/{external_type}/opportunities/{external_id}
Request Body
{
"name": "Enterprise - Rhythm",
"close_date": "2024-09-30",
"created_date": "2024-09-11",
"stage_name": "Ready to Close",
"probability": "90",
"amount": 100000.0,
"opportunity_type": "Renewal",
"account_id": "94494305",
"owner_id": "160984",
"is_closed": false,
"is_won": false,
"tags": [
"Q3"
],
"custom_fields": {
"Primary Contact": "My Champion"
}
}
Retrieving Opportunities
With External Ids: GET v2/external/ids/{external_type}/opportunities/?external_ids[]
Without External Ids: GET v2/opportunities
Query Parameters:
- external_ids[] : Provide the external ID(s) to retrieve specific opportunities.
Response Body
{
"data": [
{
"is_closed": false,
"created_date": "2024-09-11T00:00:00.000000-04:00",
"created_by_crm_id": null,
"account_name": "Revenue Orchestration",
"close_date": "2024-09-30",
"account_crm_id": null,
"id": 182450872,
"amount": "100000.00",
"most_recent_step": null,
"name": "Enterprise - Rhythm",
"most_recent_step_number": null,
"company_crm_id": null,
"created_at": "2024-09-11T09:47:12.146140-04:00",
"crm_type": null,
"opportunity_type": "Renewal",
"owner_crm_name": "Sales Rep",
"owner_crm_id": null,
"account": {
"_href": "https://api.salesloft.com/v2/accounts/94494305",
"id": 94494305
},
"opportunity_window_person_id": null,
"stage_name": "Ready to Close",
"is_won": false,
"crm_url": null,
"updated_at": "2024-09-11T10:23:27.339840-04:00",
"crm_id": null,
"custom_fields": {
"Primary Contact": "My Champion"
},
"creator": {
"_href": "https://api.salesloft.com/v2/users/160984",
"id": 160984
},
"tags": [
"Q3"
],
"opportunity_window_started_at": null,
"owner": {
"_href": "https://api.salesloft.com/v2/users/160984",
"id": 160984
},
"probability": "90.0",
"crm_last_updated_date": null,
"most_recent_cadence": null,
"currency_iso_code": null,
"most_recent_step_account": null
}
],
"metadata": {
"filtering": {
"ids": [
"182450872"
]
},
"paging": {
"current_page": 1,
"next_page": null,
"per_page": 25,
"prev_page": null
},
"sorting": {
"sort_by": "created_at",
"sort_direction": "DESC NULLS LAST"
}
}
}
Note: All CRM fields will be null for Opportunities created via API. The crm_owner_name is derived from the owner_id field on the request. If no owner_id is present on the request, this field will return the name of the user associated with the API key or auth token.
Associate with People
Add a buyer to an opportunity record. This step must be completed for Opportunity records to be shown on the Salesloft person page.
Upsert Endpoint: POST v2/opportunity_people
{
"opportunity_id": "180076055",
"person_id": "327888714"
}
Delete Opportunities
Opportunities are soft deleted. You can also delete mappings and configurations related to opportunities.
Endpoint: DELETE v2/external/ids/{external_type}/opportunities/{external_id}
View Opportunities in Salesloft
Salesloft Account & Person Page
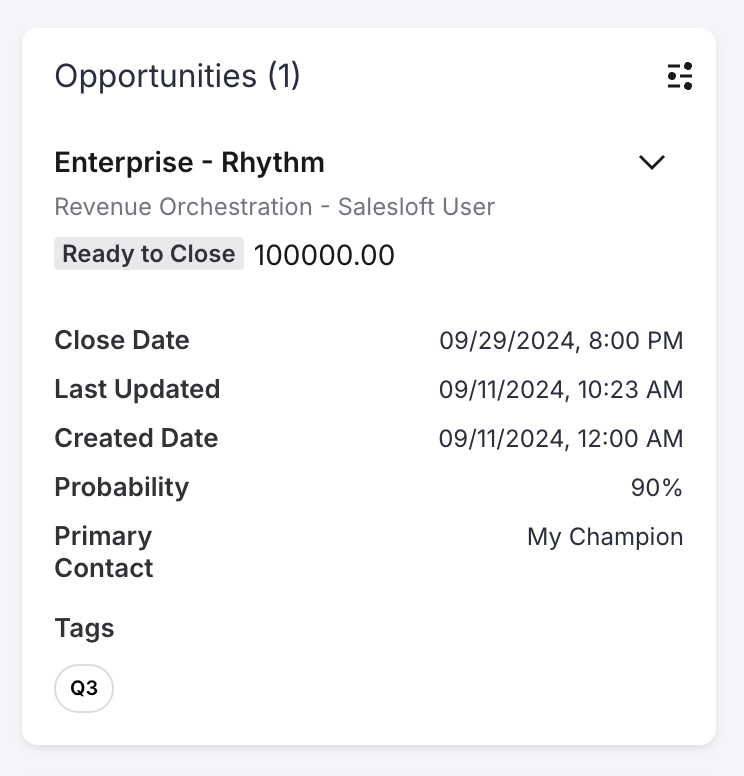
Notes
-
Error Handling: Always check for error responses (status codes 4xx and 5xx) and handle them appropriately.
-
Polling for updates: When retrieving lists of resources, implement our cursor-based polling method for the best results.